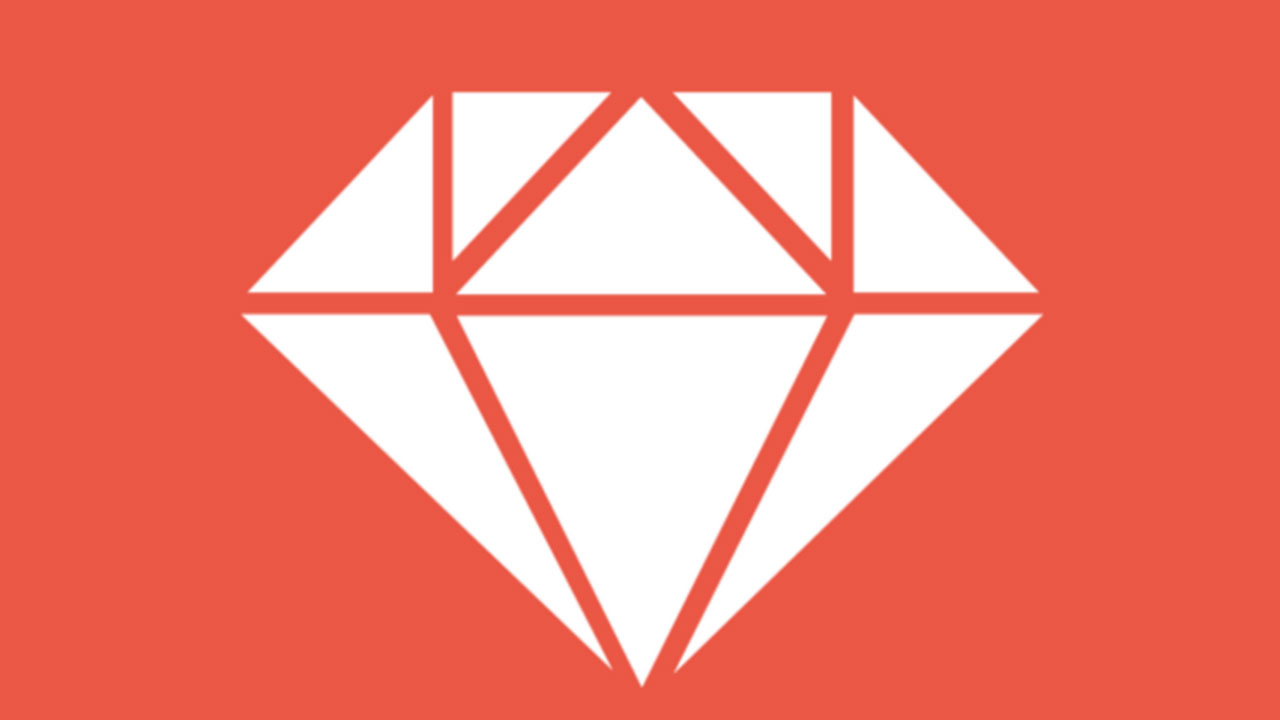
12 Sep Lambdas vs Procs vs Blocks
Blocks
I’m sure as you started learning Ruby you heard time and time again that everything is an object. That’s sort of true. but not really. A block is an anonymous function that can be passed into methods.
Blocks are enclosed in a do / end
statement or between brackets {}
, and they can have multiple arguments.
Ruby blocks are useful because they allow you to save a bit of logic (code) & use it later.
This could be something like writing data to a file, comparing if one element is equal to another, or even printing an error message.
Lambdas vs Procs
Procs and lambdas are a very similar, one of the few differences being how they’re created.
test1 = lambda{puts "your text to puts out"}
test2 = Proc.new{puts "your second text to puts out"}
alt.lambda = -> {puts "this is also a way to write a lambda"}
now let’s use .call to run them…
test1.call
puts out "your text to puts out"
But wait there’s more… ways to call a lambda
test1.()
test1[]
test1.===
Lambdas can also take arguments…
mathExample = -> (x){x*2}
mathExample.call(10)
Would return 20
“Proc” is short for “procedure,” an is many ways very similar to a method or function. If you pass the wrong number of arguments to a lambda
, it will raise an exception, just like a regular method.
But that’s not the case with procs
, as demonstrated in the following example.
test4 = Proc.new {|x,y| puts ""Doesn't care about the arguments"}
test4.call
puts out "Doesn't care about the arguments"
The big difference between procs
and lambdas
is how they react to a return
statement.
Lambdas have what are known as “diminutive returns”. What that means is that a Lambda will return flow to the function that called it, while a Proc will return out of the function that called it.
def proc_demo
Proc.new { return "return value from Proc" }.call
"return value from method"
end
def lambda_demo
lambda { return "return value from lambda" }.call
"return value from method"
end
proc_demo #=> "return value from Proc"
lambda_demo #=> "return value from method"
As you can see from the above example, the proc returns value inside the block and then ends there
but the lambda runs the content inside the block then returns the next string outside the lambda.
Also published on Medium.