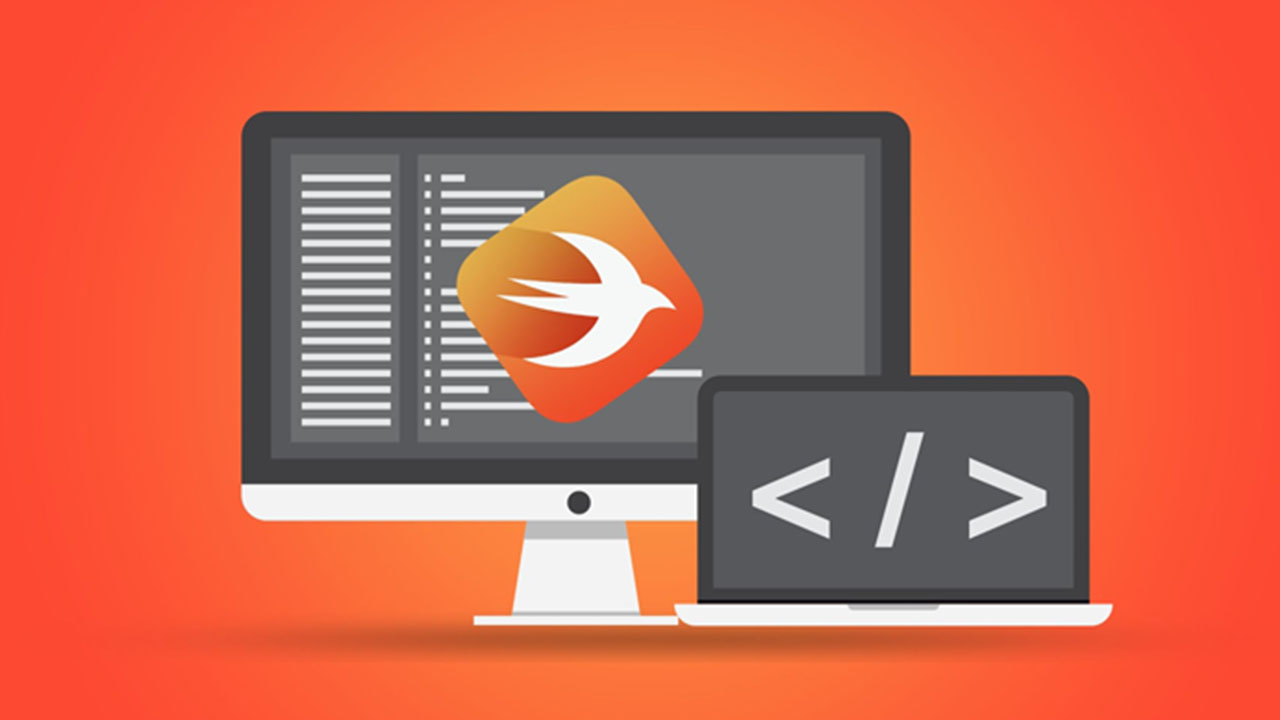
28 Aug Swift Syntax Tips
There’s seems to always be more to learn as a developer and once I do learn a new language or library I end up moving on to the next one so for me it’s really important to go back and refresh syntax. Lately, I’ve been diving back into rails but I’ve also started refreshing my basic swift skills. Let’s go back to basics and start with variables and a look at tuples.
Variables
Just like other languages constants and variables must be declared before they’re used. You declare constants with the let
keyword and variables with the var
keyword.
var str = "hello, world"
You can declare multiple constants or multiple variables on a single line, separated by commas:
var x = 5, y = 10, z = 12
Type Annotations
To be clear what type of value is stored you can:
var helloThere: String
Then you can set it as:
helloThere = "Hello"
Naming
Constant and variable names can contain almost any character, including Unicode characters:
let 🐶 = "dog"
Semicolons
Unlike many other languages, Swift doesn’t require you to write a semicolon (;) after each statement in your code, although you can do so if you wish. However, semicolons are required if you want to write multiple separate statements on a single line:
let archer = "dog"; print(archer)
Tuples
Tuples group multiple values into a single compound value. The values within a tuple can be of any type and don’t have to be of the same type as each other. You can use a tuple type as the return type of a function to enable the function to return a single tuple containing multiple values. You can also name the elements of a tuple type and use those names to refer to the values of the individual elements. An element name consists of an identifier followed immediately by a colon (:).
let mask = (5.5, "grey")
The constant mask would be of type (Int, String)
You can create tuples from any permutation of types, and they can contain as many different types as you like.
Decomposing A Tuple
You can decompose a tuple’s contents into separate constants or variables
let mask = (5.5, “grey”)
let (size, color) = mask
print(“The width of the mask is \(size)”)
prints out ” The width of the mask is 5.5″
print(“The color of the mask is \(color)”)
prints out “The color of the mask is grey”
You can access items inside the tuple just like you would with an array in JavaScript using the index:
mask.0; mask.1
You can also name the individual elements in a tuple when the tuple is defined:
let dog = (breed: “doberman”, name: “Archer”)
Once the elements have types/names you can access them using those names
dog.breed
Also published on Medium.